Sep 22, 2024 · 25 mins read
Collections in Java
Review collections in JavaUmakant Vashishtha
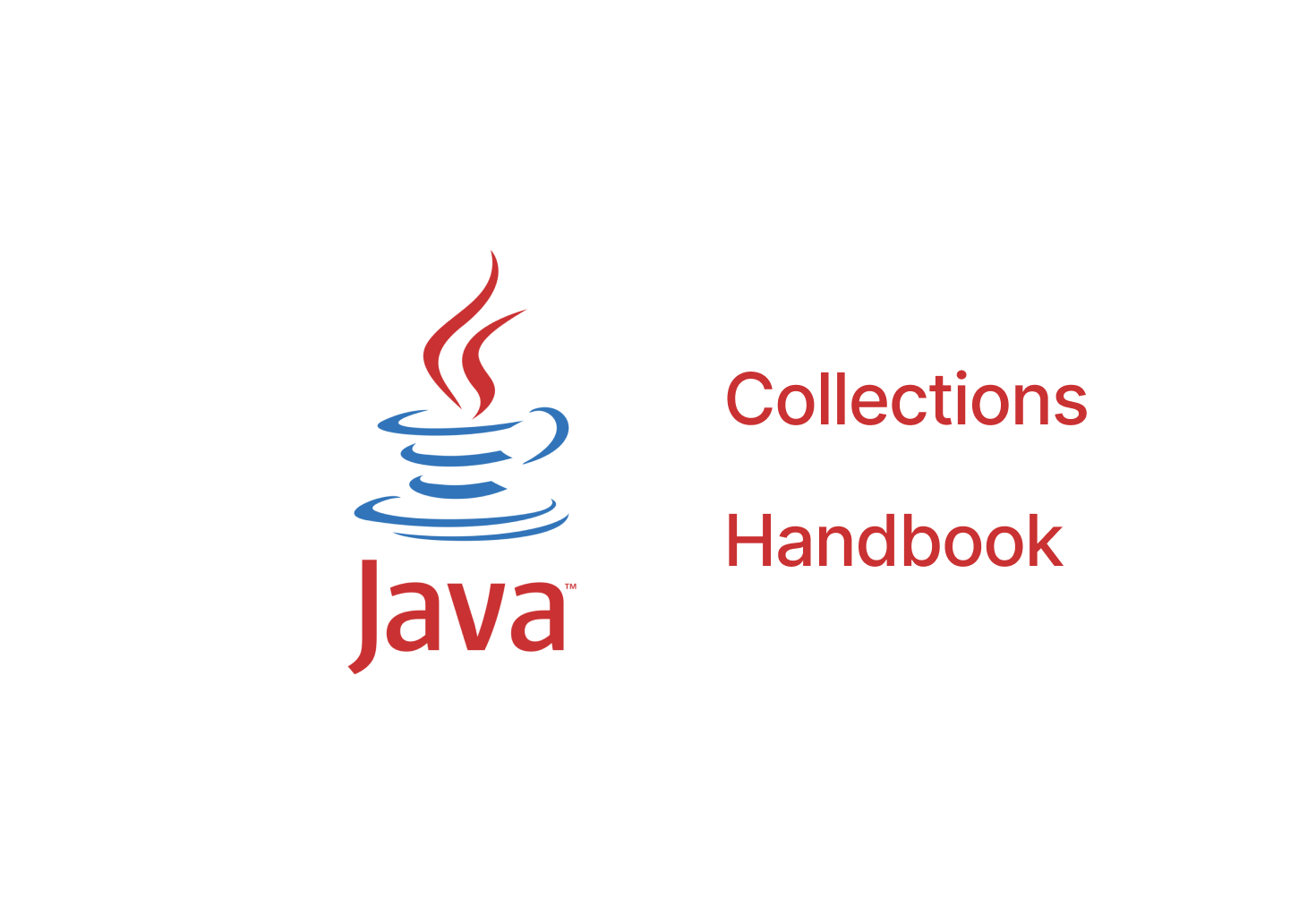
Collections in Java
Collections in Java
Lists
package com.example.collections;
import java.util.*;
class JavaCollections {
public static void main(String[] args) {
// Lists - ArrayList
List<String> strings = List.of("Java", "is", "cool");
String message = String.join(" ", strings);
System.out.println(message);
// message returned is: "Java is cool"
// ArrayList
ArrayList<String> words = new ArrayList<String>();
words.add("Name");
words.add("is");
words.add("Bond");
words.add("James Bond");
words.add("Bond");
for (String word : words) {
System.out.println(word);
}
System.out.println(words.get(3)); // James Bond
System.out.println(words.size()); // 5
System.out.println(words.contains("Bond")); // true
System.out.println(words.indexOf("Bond")); // 2
System.out.println(words.remove(3)); // James Bond
System.out.println(words.size()); // 4
System.out.println(words.remove("Bond")); // true
System.out.println(words.size()); // 3
// Join words with a space
System.out.println(String.join(" ", words)); // Name is Bond
}
}
Sorting Containers
package com.example.collections;
import java.util.*;
class JavaCollections {
public static void main(String[] args) {
// Sorting Lists
Collections.reverse(words);
Collections.reverse(words);
Collections.sort(words);
System.out.println(String.join(" ", words)); // Bond Name is
Collections.sort(words, Collections.reverseOrder());
System.out.println(String.join(" ", words)); // is Name Bond
// custom comparator
Collections.sort(words, (String a, String b) -> {
// return a.length() - b.length();
return a.charAt(a.length() - 1) - b.charAt(b.length() - 1);
});
Collections.sort(words);
int position = Collections.binarySearch(words, "abc");
System.out.println(position); // 0
System.out.println(String.join(" ", words)); // is Name Bond
}
}
Maps
package com.example.collections;
import java.util.*;
class JavaCollections {
public static void main(String[] args) {
// Maps - HashMap, TreeMap
// Unordered Map - HashMap
HashMap<String, String> map = new HashMap<String, String>();
map.put("James Bond", "007");
if (map.get("James Bond") != null) {
System.out.println(map.get("James Bond")); // 007
}
System.out.println(map.get("James Bondy")); // null
// Ordered Map - TreeMap
Map<String, String> map2 = new TreeMap<String, String>();
map2.put("008", "James Bondy");
map2.put("007", "James Bond");
for (String key : map2.keySet()) {
System.out.println(key + " " + map2.get(key));
}
}
}
Sets
package com.example.collections;
import java.util.*;
class JavaCollections {
public static void main(String[] args) {
// Sets
// Unordered Set - HashSet
Set<String> strings2 =
new HashSet<>(List.of("Java", "is", "very", "cool"));
System.out.println(strings2.contains("java"));
String message2 = String.join("-", strings2);
System.out.println(message2);
// message returned is: "Java-is-very-cool"
// Ordered Set - TreeSet
SortedSet<String> strings3 = new TreeSet<>();
strings3.add("java");
strings3.add("is");
strings3.add("very");
strings3.add("cool");
String message3 = String.join("-", strings3);
System.out.println(message3);
}
}
Queues
package com.example.collections;
import java.util.*;
class JavaCollections {
public static void main(String[] args) {
// Queues
// PriorityQueue
PriorityQueue<String> queue = new PriorityQueue<>();
queue.add("James Bond");
queue.add("007");
queue.add("Smart and cool");
System.out.println(queue.peek()); // 007 - returns the head of the queue, does not remove it
queue.remove(); // removes the head of the queue
System.out.println(queue.poll()); // James Bond - removes the head of the queue
// Deque
Deque<String> deque = new ArrayDeque<>();
deque.addLast(message3);
System.out.println(deque.peekLast());
System.out.println(deque.pollLast());
}
}
Similar Articles
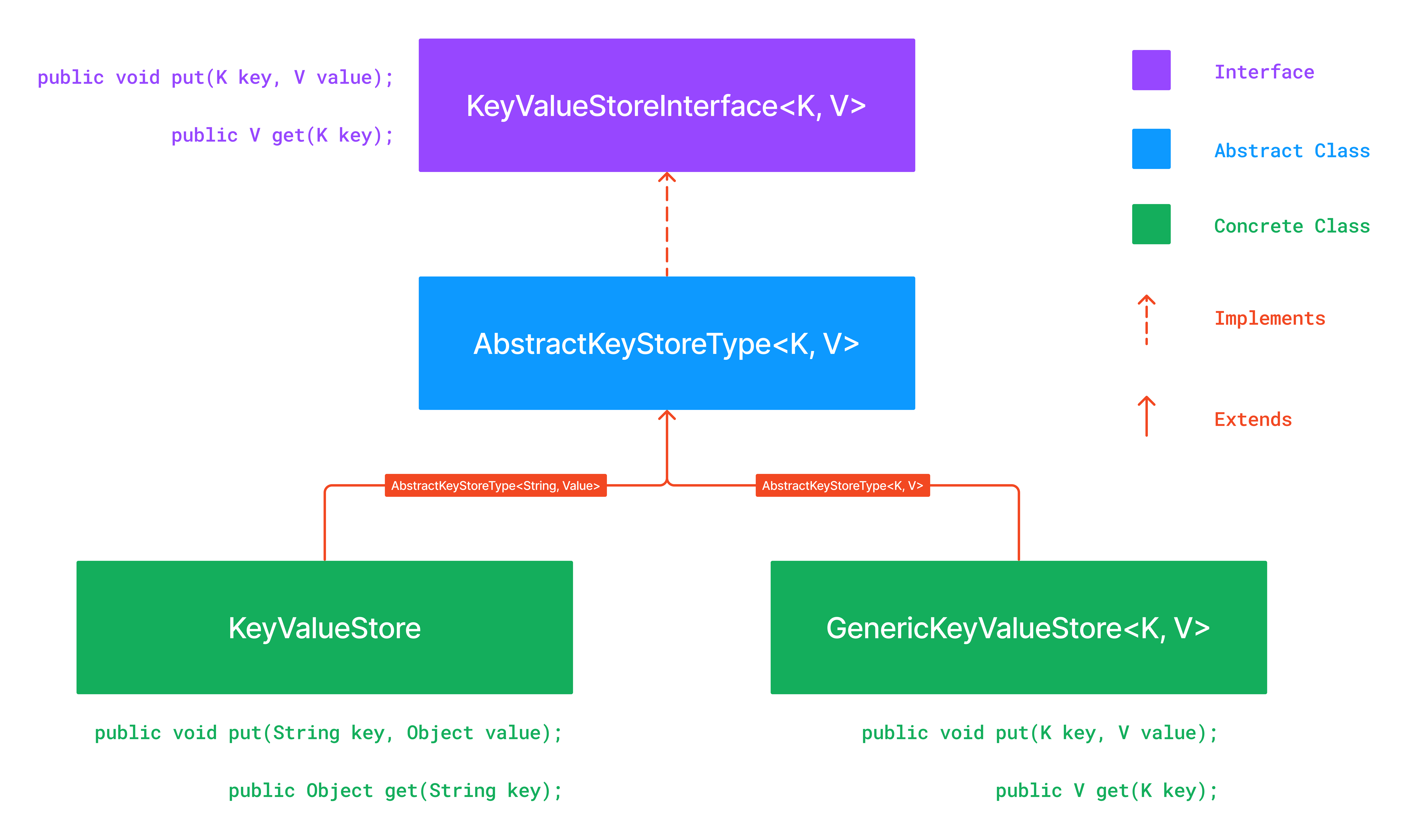
Generics in Java
Implementing generics in Java
Sep 22, 2024 · 25 mins
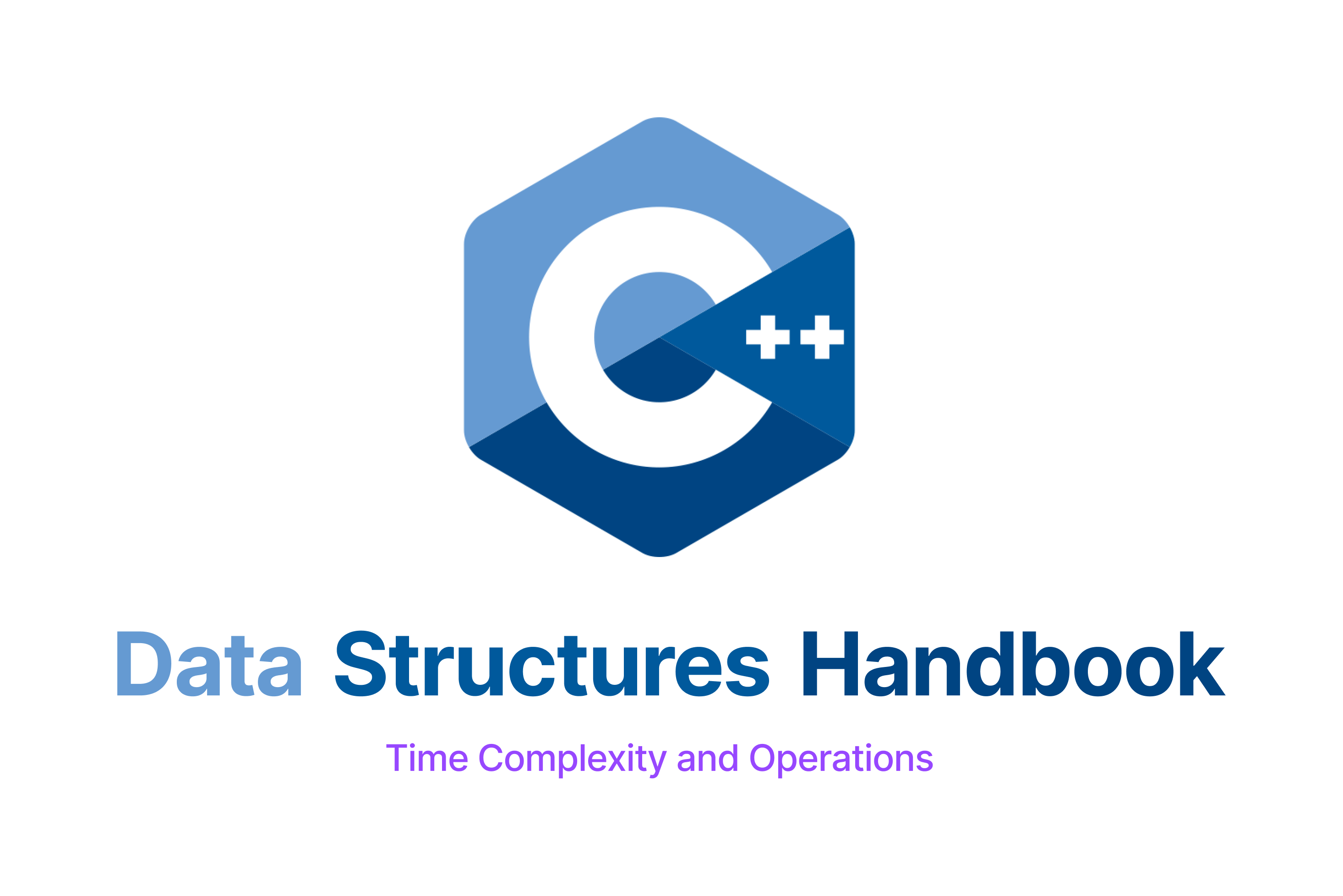
C++ Data Structures Handbook
A handbook of built-in data structures and their methods with examples and complexity analysis.
Feb 01, 2024 · 45 mins
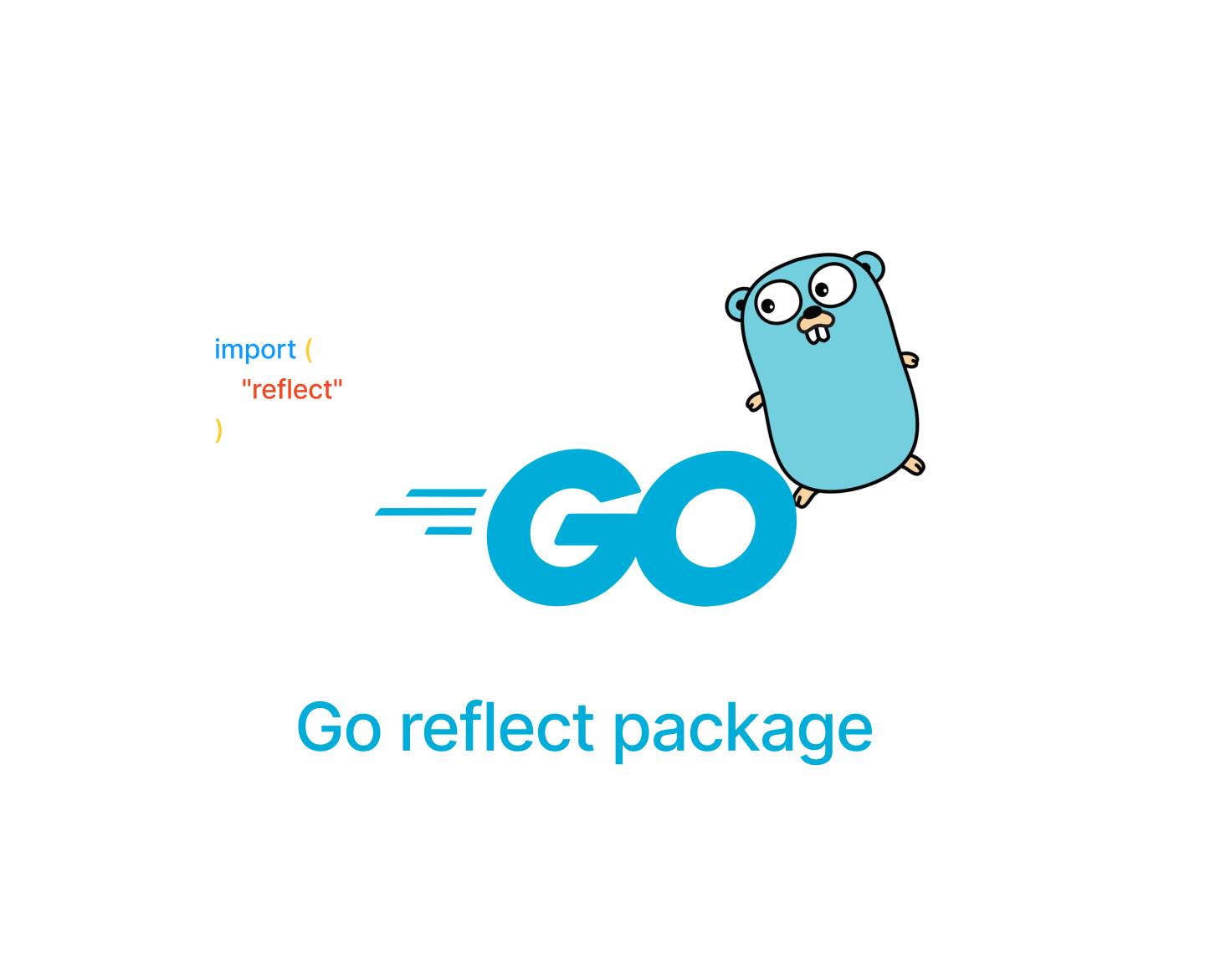
Using Reflection in Go
Using Go's reflect package to filter based on selective fields on struct
Mar 10, 2024 · 15 mins