Sep 22, 2024 · 25 mins read
Generics in Java
Implementing generics in JavaUmakant Vashishtha
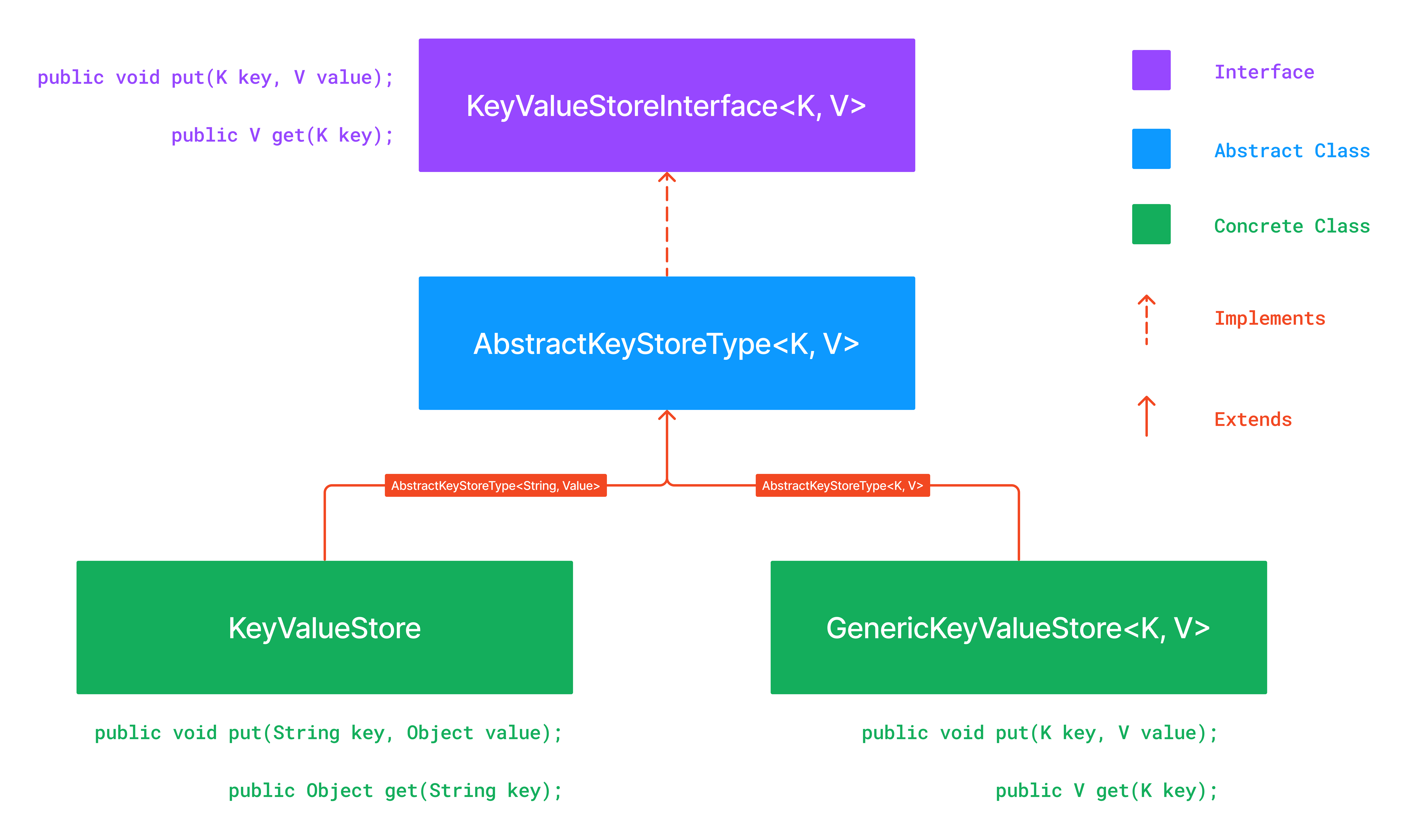
Generics in Java
Generics in Java
Generics allow you to define a class with placeholders for the type of its fields, methods, and parameters. This allows you to create a class that can be used with different types of data.
Generics are a way to implement polymorphism in Java.
We will use an example of a key-value store to understand generics in Java.
The KeyValueStore Interface
First, let’s define the most basic interface for a key-value store.
This defines the basic operations that a any key-value store should support.
/**
* KeyValueStoreInterface
*/
public interface KeyValueStoreInterface<K, V> {
/**
* This method adds a key-value pair to the store.
*/
public void put(K key, V value);
/**
* This method returns the value associated with the key.
*/
public V get(K key);
}
The Abstract Class
While the above interface defines the basic operations, we should now define an abstract class.
This class can provide a default implementation for the put
and get
methods.
public abstract class AbstractKeyStoreType<K, V>
implements KeyValueStoreInterface<K, V> {}
A Simple Implementation with String Keys
Now, let’s create a simple implementation of the key-value store with String
keys.
/**
* This class is a simple key-value store.
* It stores keys of type String and values of type Object.
*
* This class is not generic.
* It can only store keys of type String.
*/
public class KeyValueStore extends AbstractKeyStoreType<String, Object> {
HashMap<String, Object> map = new HashMap<String, Object>();
/**
* This method adds a key-value pair to the store.
*/
public void put(String key, Object value) {
map.put(key, value);
}
public Object get(String key) {
if (!map.containsKey(key)) {
return null;
}
return map.get(key);
}
}
Note how the KeyValueStore
class extends the AbstractKeyStoreType
class and provides implementations for the put
and get
methods.
While the above implementation may be useful for simple use cases, it is not generic. It can only store keys of type String
.
Let’s say we want the same functionality but with a different type of key. We can’t set an integer as the key at compile time. For supporting keys of different types, we will have to define a new class for that.
A Generic Implementation
/**
* GenericKeyValueStore stores any type of key and value.
*/
public class GenericKeyValueStore<K, V> extends AbstractKeyStoreType<K, V> {
ArrayList<K> keys = new ArrayList<K>();
ArrayList<V> values = new ArrayList<V>();
HashMap<K, V> map = new HashMap<K, V>();
public void put(K key, V value) {
map.put(key, value);
}
public V get(K key) {
if (!map.containsKey(key)) {
return null;
}
return map.get(key);
}
}
In the above implementation, we have defined a generic class GenericKeyValueStore
that can store keys and values of any type.
Using the GenericKeyValueStore
Now, let’s see how we can use the GenericKeyValueStore
class.
public class Main {
public static void main(String[] args) {
GenericKeyValueStore<Integer, String> store = new GenericKeyValueStore<Integer, String>();
store.put(1, "One");
store.put(2, "Two");
store.put(3, "Three");
System.out.println(store.get(1)); // Output: One
System.out.println(store.get(2)); // Output: Two
System.out.println(store.get(3)); // Output: Three
}
}
Similar Articles
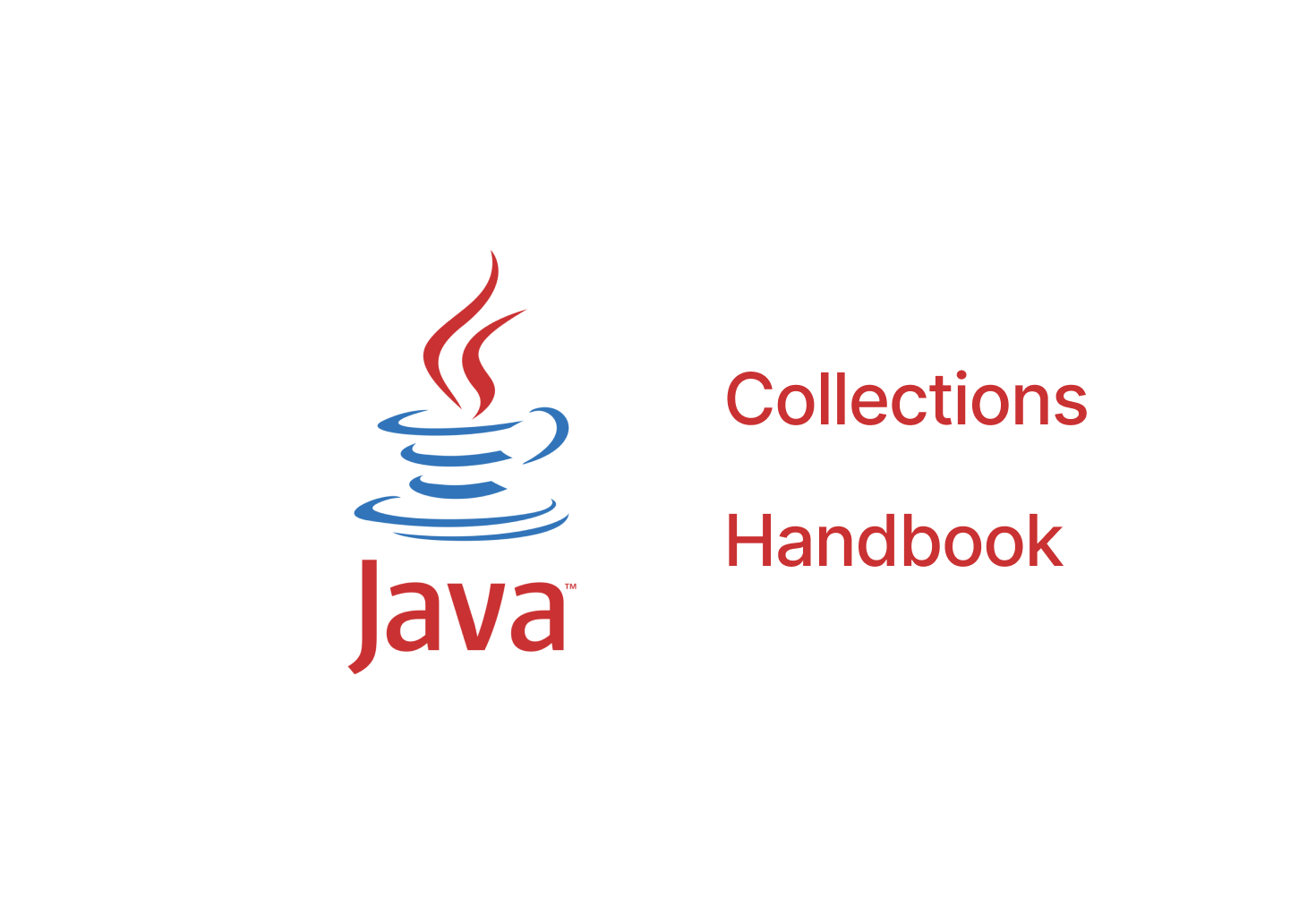
Collections in Java
Review collections in Java
Sep 22, 2024 · 25 mins
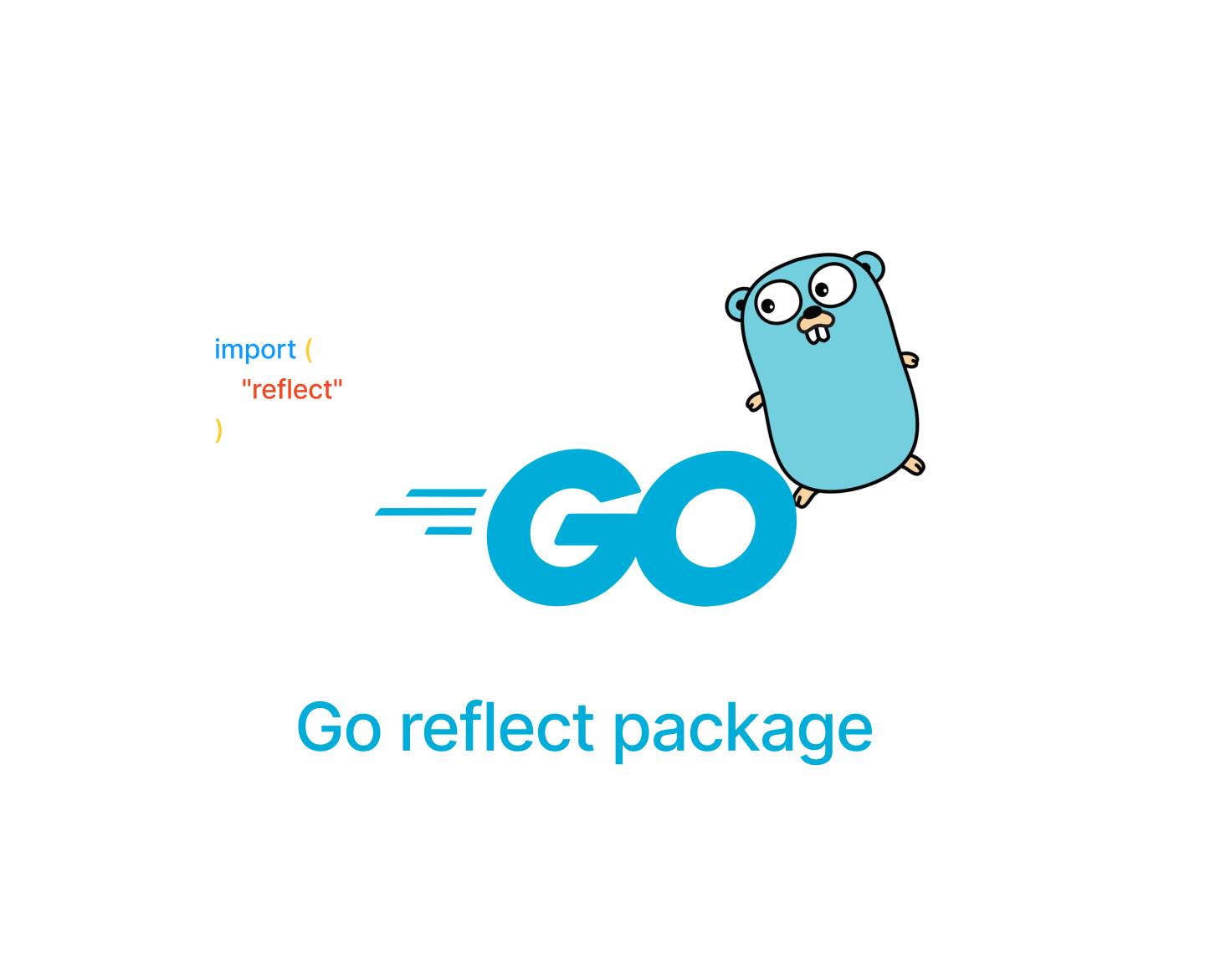
Using Reflection in Go
Using Go's reflect package to filter based on selective fields on struct
Mar 10, 2024 · 15 mins
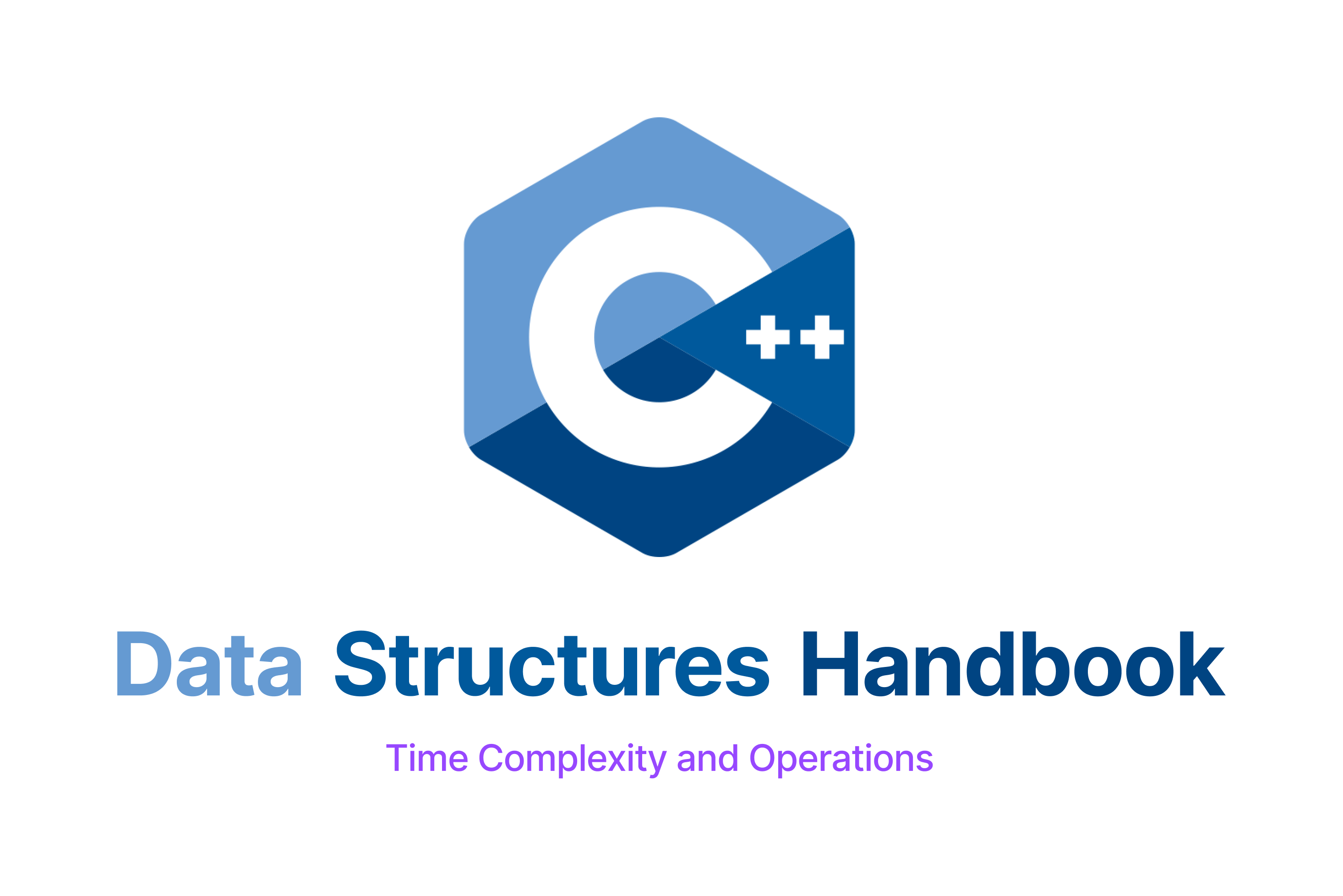
C++ Data Structures Handbook
A handbook of built-in data structures and their methods with examples and complexity analysis.
Feb 01, 2024 · 45 mins