June 20, 2023 · 40 mins read
MongoDB Operations - An Extensive Guide
Extended List of Basic MongoDB OperationsUmakant Vashishtha
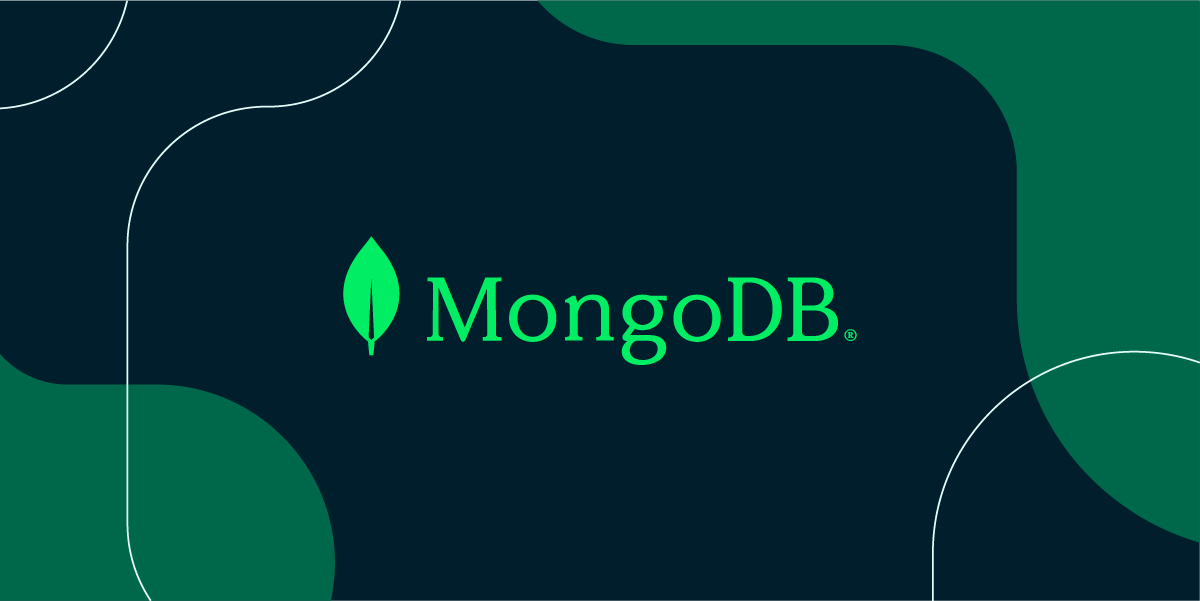
MongoDB Operations
MongoDB Operations
MongoDB Commands
Import and export Data
Run this in terminal, not in mongosh.
You would need to download these tools from here if mongodump
and mongorestore
are not available:
https://www.mongodb.com/docs/database-tools/mongorestore/
mongodump --db users-example // this will export all the data in a dump folder
mongorestore --db users-example users.bson
Show Databases
show dbs
Switch Database
use users-example
Create Collection
db.createCollection('students')
Delete a Collection
db.lectures.drop()
Show Collections
show collections
Operations
All Operators are very well documented here
Create Document in a collection
db.lectures.insertOne({ title: "Nodejs Intro", sprint: 1, date: "2023-06-08" });
Insert Many
db.students.insertMany([
{
name: "Chandler Bing",
gender: "Male",
shirt_size: "M",
language: "English",
age: 32,
friends: [
"Monika Geller",
"Ross Geller",
"Phoebe Buffay",
"Joey Tribbiani",
"Rachel Green",
"Chandler Bing",
],
},
{
name: "Monika Geller",
gender: "Female",
shirt_size: "S",
language: "English",
age: 30,
friends: [
"Ross Geller",
"Phoebe Buffay",
"Joey Tribbiani",
"Rachel Green",
"Chandler Bing",
],
},
{
name: "Ross Geller",
gender: "Male",
shirt_size: "XL",
language: "English",
age: 29,
friends: [
"Monika Geller",
"Phoebe Buffay",
"Joey Tribbiani",
"Rachel Green",
"Chandler Bing",
],
},
]);
Find Documents in a collection
db.lectures.find();
db.lectures.find({ title: "MongoDB" });
db.lectures.findOne({ _id: ObjectId("6399f881d17280baeb06c26f") });
Update Document in a collection
db.lectures.updateOne(
{ _id: ObjectId("6399f9efd17280baeb06c270") },
{
$set: { title: "Updated 2", date: "" }, // To set a field value
}
);
db.students.updateMany(
{
age: 20,
},
{
$set: {
age: 24,
graduated: true,
},
}
);
Delete Documents
db.students.deleteOne({ _id: ObjectId("6453c91019f77d5cc446f242") });
db.students.deleteMany({ name: "Test user" });
More Functions
Count
db.students.countDocuments();
db.students.countDocuments({ shirt_size: "XL" });
Sort, Limit, Skip
db.students.find({ shirt_size: "L" }).sort({
name: 1, // -1 for descending order
});
db.students.find().sort({
shirt_size: 1,
name: 1, // -1 for descending order
});
// Skipping first 2 records
db.students.find().sort({ shirt_size: 1, name: 1 }).limit(3).skip(2);
MongoDB Operators
db.students.find({
field_name1: {
operator1: value1,
operator2: value2,
},
field_name2: {
operator3: value3,
operator4: value4,
},
});
Comparison Operators
<
, >
, <=
, >=
, $ne
, $eq
db.students.find({
age: {
$lt: 60, // '<'
$gt: 40, // '>'
$gte: 41, // '>='
$lte: 59, // '<='
},
});
db.students.find({ name: { $eq: "Monika Geller" } });
db.students.find({ name: "Monika Geller" });
db.students.find({ name: { $eq: "Monika Geller" }, age: { $lt: 30 } });
$in
, $nin
$in
- All documents for which field’s values matches values in the given array will be included
$nin
- All documents for which field’s value matches values in the given array will not be included
db.students.find({
name: {
$in: ["Monika Geller", "Ross Geller", "Chandler Bing"],
},
age: {
$nin: [30, 31, 32],
},
});
Logical Operators
Used to combine different conditions
AND
, OR
, NOT
, NOR
db.students.find({
$logical_operator: [
{
// $condition1
name: {},
age: {},
shirt_size: {},
},
{
// $condition2
name: {},
age: {},
shirt_size: {},
},
{
// $condition3
name: {},
age: {},
shirt_size: {},
},
],
});
db.students.find({
$and: [
{
name: {
$in: ["Monika Geller", "Ross Geller", "Chandler Bing"],
},
},
{
age: {
$nin: [30, 31, 32],
},
},
],
});
db.students.find({
name: {
$in: ["Monika Geller", "Ross Geller", "Chandler Bing"],
},
age: {
$nin: [30, 31, 32],
},
});
db.students.find({
$or: [
{
name: {
$in: ["Monika Geller", "Chandler Bing"],
},
},
{
age: {
$in: [29, 31, 32],
},
},
],
});
db.students.find({
age: {
$not: {
$in: [29, 30, 22],
},
},
});
db.students.find({
age: {
$not: {
$lt: 29,
},
},
});
db.students.find({
$nor: [
// MUST FAIL ALL CONDITIONS
{
name: {
$in: ["Monika Geller", "Ross Geller", "Chandler Bing"],
},
},
{
age: {
$in: [30, 31, 32],
},
},
],
});
Array Operators
$all
, $size
, $elemMatch
$all
- All the given values must be present in the array in the document$size
- Size of the array in the document must be equal to the given number$elemMatch
- If any element in the array in the document which matches this condition is present
db.students.find({
friends: {
$all: ["Ross Geller", "Chandler Bing"],
},
});
db.students.find({
friends: {
$size: 6,
},
});
db.students.find({
friends: {
$elemMatch: {
//
$lt: "Z",
$gt: "P",
},
},
});
Element Operators
$exists
, $type
$exists
- Whether the given field exists in the document$type
- Field must exist in the document and the type of the field should match the given type
db.students.find({
friends: {
$exists: true, // field must exists
},
});
db.students.find({
age: {
$exists: true,
$type: "number",
},
});
db.students.find({ graduated: { $exists: true, $type: "bool" } });
Evaluation Operators
$mod
, $regex
, $text
$mod
- Divide the numeric value of the field in the document with a given number (except 0) and expect the given remainder$regex
- Match the regulaer expression on the string values$text
- Fuzzy Search
db.students.find({
age: {
$mod: [4, 1], // when age is divided by 4, remainder should be 1
},
});
db.students.find({
name: {
$regex: /^Mon/,
},
});
db.students.createIndex({ name: "text" });
db.students.find({
$text: {
$search: "Monika Bing",
},
});
// title_text index would be created
// This index can be deleted
Fuzzy Search Example
db.posts.createIndex({ title: "text", content: "text" });
// title_text_content_text
db.posts.insertMany([
{
title: "Nodejs Intro",
content: "Introduction to Nodejs",
},
{
title: "MongoDb Intro",
content: "Introduction to Mongodb",
},
{
title: "Graphql Intro",
content: "Introduction to Express",
},
{
title: "Auth Intro",
content: "Introduction to OAuth",
},
]);
db.posts.find({
$text: {
$search: "intro graphql",
},
});
db.posts.find({
$text: {
$search: "course graphql",
},
});
Similar Articles
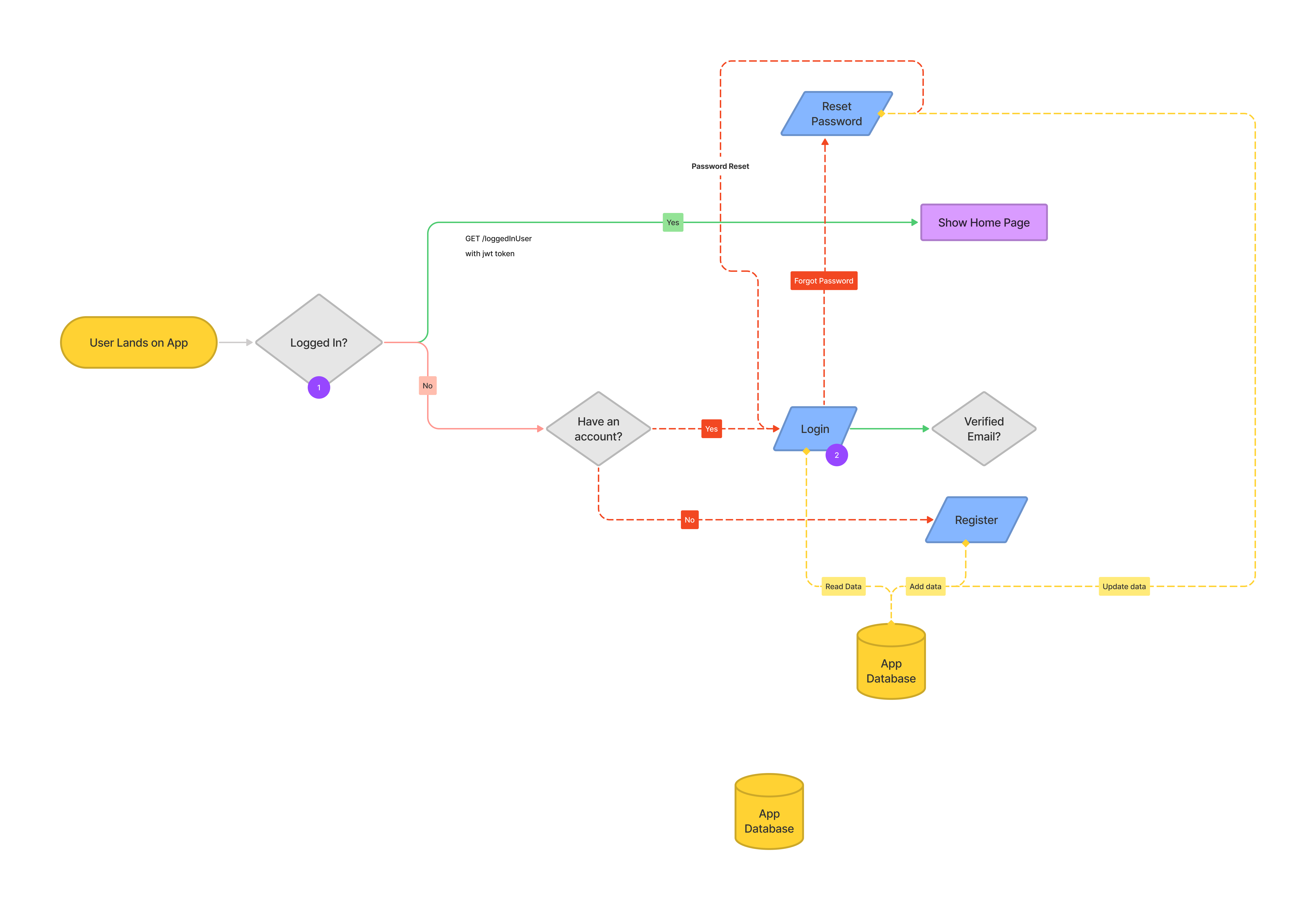
Authentication — A Step-by-Step Explanation
Authentication — A Step-by-Step Based Explanation | by Umakant Vashishtha | Jun, 2023
Jun 26, 2023 · 8 mins
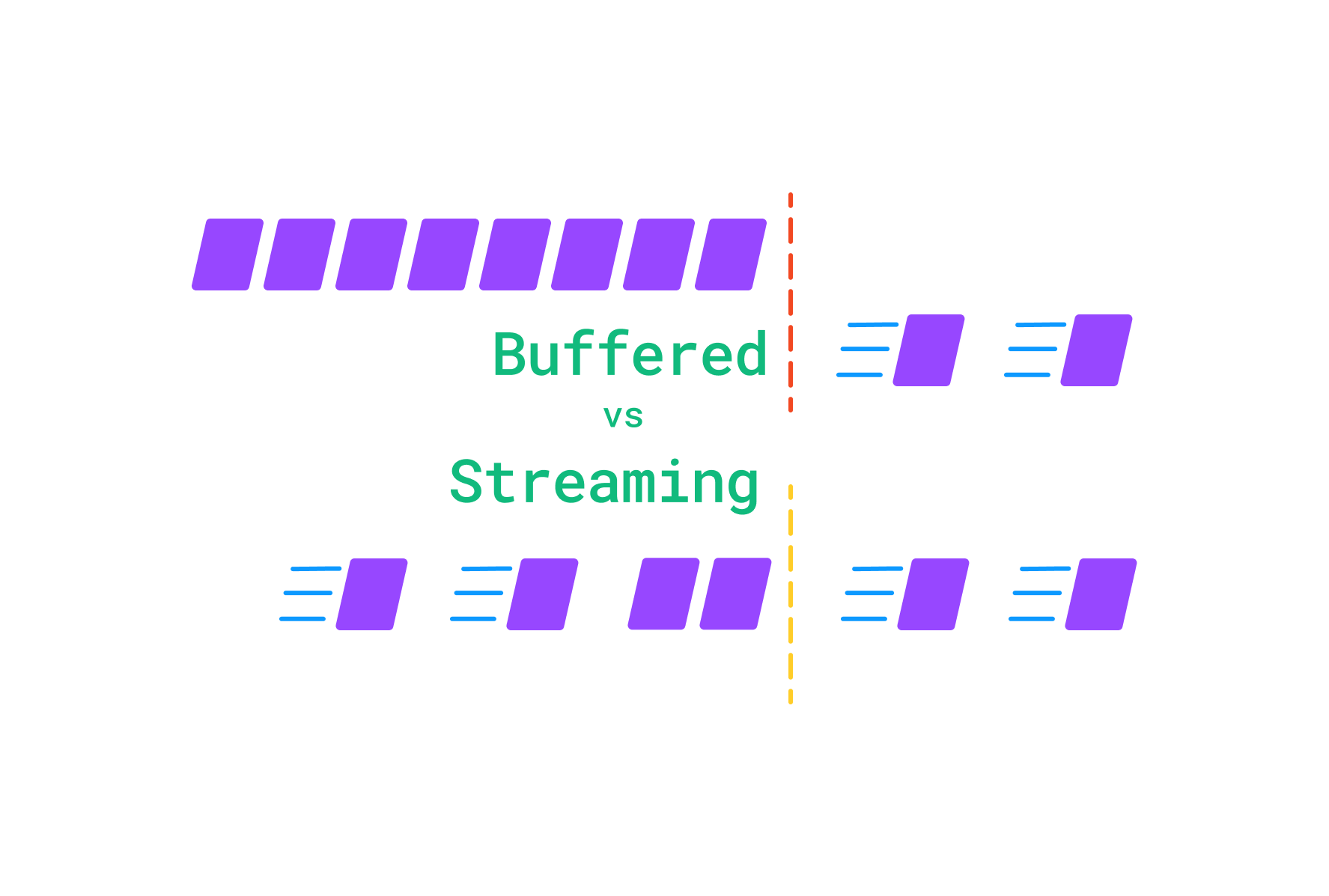
Buffered vs Streaming Data Transfer
Comparing buffered and streaming data transfer in Node.js server
Oct 27, 2023 · 15 mins
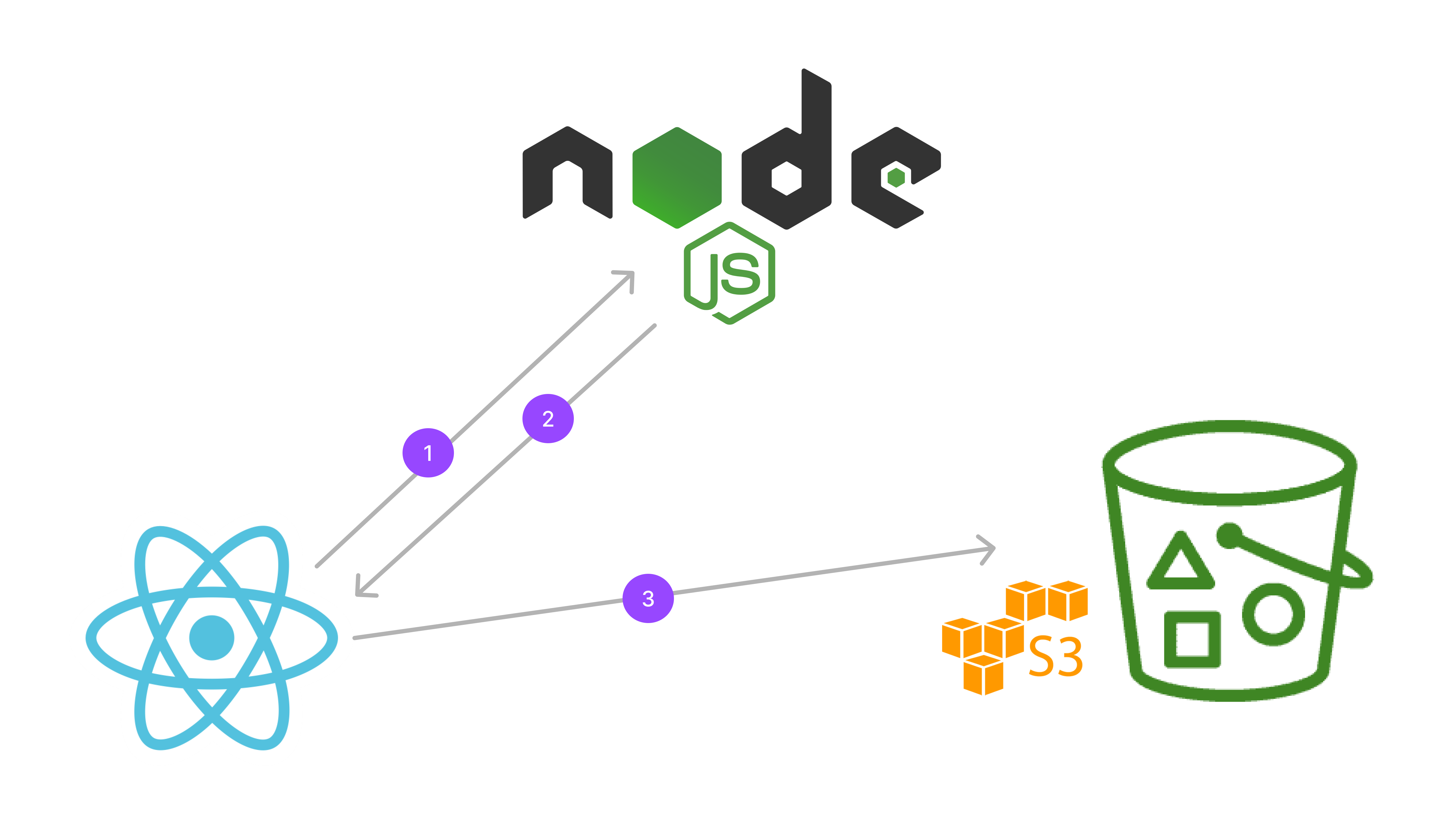
Using Signed URLs in React | File Upload using AWS S3, Node.js and React - Part 3
Building the react application to upload files directly to AWS S3 using Signed URLs generated from our node.js application.
Oct 15, 2023 · 15 mins